How Developers Can Improve Their Debugging Techniques By Gustavo Woltmann
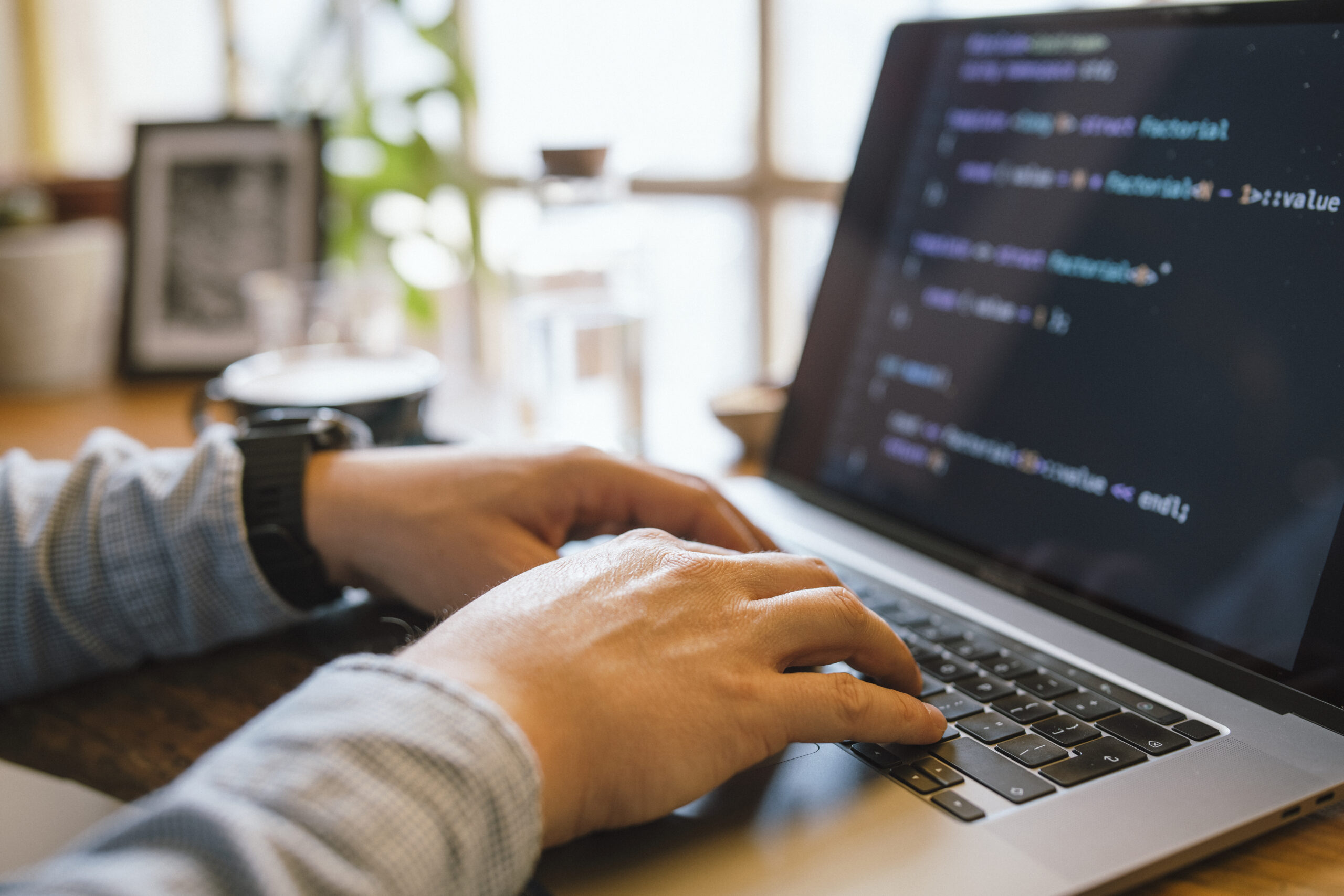
Debugging is Among the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of annoyance and considerably transform your productiveness. Allow me to share many techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Grasp Your Equipment
On the list of fastest techniques developers can elevate their debugging skills is by mastering the applications they use everyday. When producing code is one particular Section of advancement, understanding how to connect with it efficiently throughout execution is Similarly vital. Modern-day growth environments come Geared up with effective debugging capabilities — but lots of developers only scratch the floor of what these resources can perform.
Just take, for instance, an Built-in Advancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When used accurately, they let you notice exactly how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can change disheartening UI problems into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Learning these resources could possibly have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate units like Git to know code background, uncover the precise minute bugs were being released, and isolate problematic alterations.
In the long run, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your improvement surroundings to ensure when difficulties occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time you may commit fixing the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most crucial — and often overlooked — ways in helpful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the precise conditions underneath which the bug happens.
When you’ve gathered sufficient facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the identical data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply help expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue could possibly be ecosystem-certain — it would materialize only on particular working devices, browsers, or under specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can continually recreate the bug, you happen to be now midway to correcting it. Which has a reproducible scenario, you can use your debugging tools much more efficiently, check prospective fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete obstacle — and that’s exactly where developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Instead of looking at them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Many builders, particularly when less than time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste error messages into search engines like yahoo — browse and recognize them initial.
Crack the error down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can manual your investigation and position you towards the accountable code.
It’s also practical to comprehend the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can greatly speed up your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to look at the context during which the mistake happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it provides true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase with the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic info during development, Facts for normal gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine troubles, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and decelerate your process. Give attention to key situations, condition adjustments, enter/output values, and significant choice details with your code.
Format your log messages Plainly and regularly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you can decrease the time it will require to identify concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To effectively recognize and correct bugs, builders will have to method the process just like a detective fixing a thriller. This mentality helps break down complicated concerns into manageable sections and observe clues logically to uncover the foundation cause.
Start by gathering evidence. Look at the signs of the trouble: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much suitable information as you could without jumping to conclusions. Use logs, check instances, and user reviews to piece together a clear picture of what’s happening.
Following, kind hypotheses. Check with on your own: What may very well be resulting in this habits? Have any alterations not long ago been designed to your codebase? Has this challenge happened before under similar instances? The target will be to slim down prospects and recognize possible culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled ecosystem. When you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, request your code concerns and Enable the final results lead you nearer to the truth.
Pay shut focus to compact information. Bugs frequently disguise while in the least anticipated places—just like a missing semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out absolutely knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed difficulties in complex techniques.
Produce Checks
Writing exams is one of the best solutions to improve your debugging abilities and Total progress performance. Checks not only assist catch bugs early but in addition serve as a safety Internet that provides you self esteem when creating adjustments in your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where to glimpse, noticeably lessening enough time put in debugging. Unit tests are Primarily practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, combine integration tests and end-to-close assessments into your workflow. These aid make sure that many portions of your application work jointly easily. They’re especially practical for catching bugs that arise in sophisticated systems with many elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline unsuccessful and beneath what circumstances.
Crafting exams also forces you to definitely Believe critically regarding your code. To test a element effectively, you need to grasp its inputs, expected outputs, and edge situations. This level of comprehension Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing test that reproduces the bug could be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you capture extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—observing your display screen for several hours, seeking solution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The problem from the new point of view.
When you are way too near to the code for also prolonged, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind results in being a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Several developers report getting the foundation of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially throughout longer debugging classes. Sitting before a display screen, mentally Gustavo Woltmann coding stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to maneuver all around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, getting breaks is not a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code assessments, or logging? The responses usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the very same concern boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your development journey. In spite of everything, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you deal with adds a fresh layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.